5. MJO
This notebook demonstrates the basic usage of the Madden-Julian Oscillation (MJO) metrics driver.
Before running this demo, please ensure that the sample data has been downloaded by running the Demo 0: Download Data notebook, if you haven’t already.
Scientific information about the MJO metrics is available through the README.
References:
Lee, J., P. J. Gleckler, M.-S. Ahn, A. Ordonez, P. Ullrich, K. R. Sperber, K. E. Taylor, Y. Y. Planton, E. Guilyardi, P. Durack, C. Bonfils, M. D. Zelinka, L.-W. Chao, B. Dong, C. Doutriaux, C. Zhang, T. Vo, J. Boutte, M. F. Wehner, A. G. Pendergrass, D. Kim, Z. Xue, A. T. Wittenberg, and J. Krasting, 2024: Systematic and Objective Evaluation of Earth System Models: PCMDI Metrics Package (PMP) version 3. Geoscientific Model Development, 17, 3919–3948, doi: 10.5194/gmd-17-3919-2024.
Ahn, M.-S., D. Kim, K. R. Sperber, I.-S. Kang, E. Maloney, D. Waliser, H. Hendon, 2017: MJO simulation in CMIP5 climate models: MJO skill metrics and process-oriented diagnosis. Clim. Dynam., 49, 4023-4045. doi: 10.1007/s00382-017-3558-4.
First we load the demo directory choices (read from user_choices.py
file in the current directory, which is generated when running the Demo 0):
[1]:
from user_choices import demo_data_directory, demo_output_directory
The --help
flag is available to provide more information on the mjo_metrics_driver:
[2]:
%%bash
mjo_metrics_driver.py --help
usage: mjo_metrics_driver.py [-h] [--parameters PARAMETERS]
[--diags OTHER_PARAMETERS [OTHER_PARAMETERS ...]]
[--results_dir RESULTS_DIR]
[--reference_data_path REFERENCE_DATA_PATH]
[--modpath MODPATH] [--mip MIP] [--exp EXP]
[--frequency FREQUENCY] [--realm REALM]
[--reference_data_name REFERENCE_DATA_NAME]
[--reference_data_lf_path REFERENCE_DATA_LF_PATH]
[--modpath_lf MODPATH_LF] [--varOBS VAROBS]
[--varModel VARMODEL]
[--ObsUnitsAdjust OBSUNITSADJUST]
[--ModUnitsAdjust MODUNITSADJUST] [--units UNITS]
[--osyear OSYEAR] [--msyear MSYEAR]
[--oeyear OEYEAR] [--meyear MEYEAR]
[--seasons SEASONS [SEASONS ...]]
[--modnames MODNAMES [MODNAMES ...]]
[-r REALIZATION] [--case_id CASE_ID] [-d DEBUG]
[--nc_out NC_OUT] [--plot PLOT]
[--update_json UPDATE_JSON] [--cmec] [--no_cmec]
[--parallel] [--includeOBS] [--no_OBS]
[--num_workers NUM_WORKERS]
Runs PCMDI MJO Computations
options:
-h, --help show this help message and exit
--parameters PARAMETERS, -p PARAMETERS
--diags OTHER_PARAMETERS [OTHER_PARAMETERS ...]
Path to other user-defined parameter file.
--results_dir RESULTS_DIR, --rd RESULTS_DIR
The name of the folder where all runs will be stored.
--reference_data_path REFERENCE_DATA_PATH, --rdp REFERENCE_DATA_PATH
The path/filename of reference (obs) data.
--modpath MODPATH, --mp MODPATH
Explicit path to model data
--mip MIP A WCRP MIP project such as CMIP3 and CMIP5
--exp EXP An experiment such as amip, historical or piContorl
--frequency FREQUENCY
--realm REALM
--reference_data_name REFERENCE_DATA_NAME
Name of reference data set
--reference_data_lf_path REFERENCE_DATA_LF_PATH
Path of landsea mask for reference data set
--modpath_lf MODPATH_LF
Path of landsea mask for model data set
--varOBS VAROBS Name of variable in reference data
--varModel VARMODEL Name of variable in model(s)
--ObsUnitsAdjust OBSUNITSADJUST
For unit adjust for OBS dataset. For example:
- (True, 'divide', 100.0) # Pa to hPa
- (True, 'subtract', 273.15) # degK to degC
- (False, 0, 0) # No adjustment (default)
--ModUnitsAdjust MODUNITSADJUST
For unit adjust for model dataset. For example:
- (True, 'divide', 100.0) # Pa to hPa
- (True, 'subtract', 273.15) # degK to degC
- (False, 0, 0) # No adjustment (default)
--units UNITS Final units for the variable
--osyear OSYEAR Start year for reference data set
--msyear MSYEAR Start year for model data set
--oeyear OEYEAR End year for reference data set
--meyear MEYEAR End year for model data set
--seasons SEASONS [SEASONS ...]
List of seasons. Available: 'DJFMA', 'MJJASO'
--modnames MODNAMES [MODNAMES ...]
List of models. 'all' for every available models
-r REALIZATION, --realization REALIZATION
Consider all accessible realizations as idividual
- r1i1p1: default, consider only 'r1i1p1' member
Or, specify realization, e.g, r3i1p1'
- *: consider all available realizations
--case_id CASE_ID version as date, e.g., v20191116 (yyyy-mm-dd)
-d DEBUG, --debug DEBUG
Option for debug: False (defualt) or True
--nc_out NC_OUT Option for generate netCDF file output: True (default) / False
--plot PLOT Option for generate individual plots: True (default) / False
--update_json UPDATE_JSON
Option for update existing JSON file: True (i.e., update) (default) / False (i.e., overwrite)
--cmec Option to save metrics in CMEC format
--no_cmec Option to not save metrics in CMEC format
--parallel Turn on the parallel mode for running code using multiple CPUs
--includeOBS include observation
--no_OBS include observation
--num_workers NUM_WORKERS
Start year for model data set
Basic Use
The MJO driver can read a parameter file to load user settings. A basic parameter file is shown here:
[3]:
with open("basic_mjo_param.py") as f:
print(f.read())
import os
#
# OPTIONS ARE SET BY USER IN THIS FILE AS INDICATED BELOW BY:
#
#
case_id = 'Ex1'
realization = 'r6i1p1'
# ROOT PATH FOR MODELS CLIMATOLOGIES
modnames = ['GISS-E2-H']
modpath = 'demo_data/CMIP5_demo_timeseries/historical/atmos/day/pr/pr_day_%(model)_historical_r6i1p1_20000101-20051231.nc'
varModel = 'pr'
ModUnitsAdjust = (True, 'multiply', 86400.0, 'mm d-1') # kg m-2 s-1 to mm day-1
units = 'mm/d'
msyear = 2000
meyear = 2002
seasons = ['NDJFMA', 'MJJASO']
# ROOT PATH FOR OBSERVATIONS
reference_data_name = 'GPCP-IP'
reference_data_path = 'demo_data/obs4MIPs_PCMDI_daily/NASA-JPL/GPCP-1-3/day/pr/gn/latest/pr_day_GPCP-1-3_PCMDI_gn_19961002-20170101.nc'
varOBS = 'pr'
ObsUnitsAdjust = (True, 'multiply', 86400.0, 'mm d-1') # kg m-2 s-1 to mm day-1
osyear = 1998
oeyear = 1999
# DIRECTORY WHERE TO PUT RESULTS
results_dir = 'demo_output/mjo/%(case_id)'
# MISCELLANEOUS
nc_out = False
plot = True # Create map graphics
update_json = False
This parameter file can be passed to the mjo driver via the command line:
mjo_metrics_driver.py -p basic_mjo_param.py
This example is run as a subprocess in the next cell.
[4]:
%%bash
mjo_metrics_driver.py -p basic_mjo_param.py --debug True
includeOBS: True
models: ['GISS-E2-H']
realization: r6i1p1
output directories:
graphics : demo_output/mjo/Ex1
diagnostic_results : demo_output/mjo/Ex1
metrics_results : demo_output/mjo/Ex1
CMEC: False
debug: True
parallel: False
----- obs ---------------------
--- GPCP-IP ---
demo_data/obs4MIPs_PCMDI_daily/NASA-JPL/GPCP-1-3/day/pr/gn/latest/pr_day_GPCP-1-3_PCMDI_gn_19961002-20170101.nc
-- NDJFMA --
debug: open file: demo_data/obs4MIPs_PCMDI_daily/NASA-JPL/GPCP-1-3/day/pr/gn/latest/pr_day_GPCP-1-3_PCMDI_gn_19961002-20170101.nc
debug: check time
debug: startYear, endYear: 1998 1999
debug: NL, NT: 144 180
debug: before year loop: daSeaCyc.shape: (180, 180, 360)
1998
Converting units by multiply 86400.0
debug: year, segment[year][data_var].shape: 1998 (180, 180, 360)
debug: after year loop: daSeaCyc.shape: (180, 180, 360)
debug: year loop start
chk: year: 1998
debug: ds_regrid_subset[data_var] shape: (180, 8, 144)
debug: compute space-time spectrum
OEE: <xarray.DataArray 'power' (zonalwavenumber: 11, frequency: 21)> Size: 2kB
array([[2.36910155e-03, 1.51265210e-02, 1.18739458e-02, 5.81161638e-03,
1.09830500e-02, 3.86928395e-03, 2.14416805e-03, 6.52917233e-03,
7.07560778e-03, 1.00309803e-02, 1.72325645e-03, 1.00309803e-02,
7.07560778e-03, 6.52917233e-03, 2.14416805e-03, 3.86928395e-03,
1.09830500e-02, 5.81161638e-03, 1.18739458e-02, 1.51265210e-02,
2.36910155e-03],
[6.76338315e-04, 1.83563496e-02, 3.45146917e-02, 6.74832770e-03,
1.81981936e-03, 1.70161928e-03, 2.96769991e-03, 8.84541268e-03,
3.23222820e-03, 4.41311440e-02, 4.91307731e-05, 1.45325759e-02,
7.52461145e-03, 4.30240226e-02, 5.40665900e-02, 7.44683041e-03,
1.03330797e-02, 2.99916358e-02, 1.05103023e-02, 2.55793002e-02,
5.17013345e-03],
[7.27431391e-03, 1.19201516e-02, 1.78900079e-02, 3.08577963e-03,
9.87644521e-03, 4.45900288e-04, 1.32125728e-02, 1.37242942e-02,
4.18005179e-03, 1.80659496e-03, 2.42318412e-04, 1.13157799e-02,
7.62902212e-03, 7.89011934e-04, 5.12348651e-03, 6.78978981e-03,
2.72573875e-02, 2.91306810e-02, 1.73124011e-03, 1.10582104e-02,
1.52611855e-02],
[1.73798501e-03, 9.34147546e-03, 5.01560228e-03, 3.22126950e-03,
3.87026649e-03, 9.81667141e-03, 1.44738178e-03, 4.25091598e-05,
...
1.40514039e-03, 6.80917483e-04, 1.09931305e-03, 1.30763266e-03,
2.68237799e-03],
[2.97364870e-03, 3.76882823e-03, 1.49080137e-04, 4.11393259e-04,
2.84524633e-03, 9.98019537e-04, 2.75820340e-04, 3.99225284e-03,
6.86621160e-03, 1.83329828e-03, 3.92054558e-04, 5.50241078e-03,
1.97972992e-03, 3.75883174e-04, 8.41614999e-04, 2.83386424e-03,
2.74953637e-03, 4.87153083e-03, 6.70786834e-04, 1.90195272e-03,
3.78144418e-03],
[1.21214996e-05, 9.66304428e-04, 9.26323304e-04, 4.48698192e-03,
8.90605606e-04, 3.62311193e-05, 3.00316967e-04, 7.91572265e-04,
4.09180258e-04, 1.50752530e-03, 5.63113947e-04, 3.37306368e-03,
7.22179810e-05, 5.13499723e-05, 3.16019678e-03, 7.53727326e-04,
9.13666688e-05, 5.21764319e-04, 9.67519360e-04, 1.94941967e-03,
2.14967914e-03],
[9.76033329e-04, 7.81640969e-04, 6.96038209e-03, 1.40803352e-03,
3.60859518e-03, 1.02872093e-03, 3.81870585e-06, 2.70593758e-04,
1.23662802e-03, 1.70441680e-03, 2.65195130e-04, 8.58281940e-04,
3.11981369e-03, 5.42573427e-04, 8.72481664e-04, 1.58997452e-03,
9.45615999e-04, 2.57441323e-04, 9.25116598e-04, 5.45678800e-03,
1.51478898e-03]])
Coordinates:
* frequency (frequency) float64 168B -0.05556 -0.05 ... 0.05 0.05556
* zonalwavenumber (zonalwavenumber) float64 88B 0.0 1.0 2.0 ... 8.0 9.0 10.0
OEE.shape: (11, 21)
ewr: 2.933687364115082
east power: 0.016568144258499968
west power: 0.0056475493814241474
type(segment_year) <class 'xarray.core.dataarray.DataArray'>
segment_year.shape: (180, 180, 360)
type(daSeaCyc) <class 'xarray.core.dataarray.DataArray'>
daSeaCyc.shape: (180, 180, 360)
type(segment_ano_year) <class 'xarray.core.dataarray.DataArray'>
segment_ano_year.shape: (180, 180, 360)
-- MJJASO --
debug: open file: demo_data/obs4MIPs_PCMDI_daily/NASA-JPL/GPCP-1-3/day/pr/gn/latest/pr_day_GPCP-1-3_PCMDI_gn_19961002-20170101.nc
debug: check time
debug: startYear, endYear: 1998 1999
debug: NL, NT: 144 180
debug: before year loop: daSeaCyc.shape: (180, 180, 360)
1998
Converting units by multiply 86400.0
debug: year, segment[year][data_var].shape: 1998 (180, 180, 360)
debug: after year loop: daSeaCyc.shape: (180, 180, 360)
debug: year loop start
chk: year: 1998
debug: ds_regrid_subset[data_var] shape: (180, 8, 144)
debug: compute space-time spectrum
OEE: <xarray.DataArray 'power' (zonalwavenumber: 11, frequency: 21)> Size: 2kB
array([[3.89178303e-04, 1.36427559e-03, 2.39908917e-04, 6.63884694e-04,
1.29732316e-03, 1.35521970e-03, 6.10000806e-05, 9.68272687e-04,
6.11418059e-04, 4.90337391e-03, 1.11859899e-03, 4.90337391e-03,
6.11418059e-04, 9.68272687e-04, 6.10000806e-05, 1.35521970e-03,
1.29732316e-03, 6.63884694e-04, 2.39908917e-04, 1.36427559e-03,
3.89178303e-04],
[5.60566177e-04, 6.57301438e-04, 1.55138802e-04, 2.89633340e-04,
3.06136221e-04, 3.47677265e-04, 5.72217362e-04, 1.98912958e-04,
3.56935402e-04, 3.65104771e-03, 2.67765996e-05, 3.43633828e-03,
2.22699153e-03, 1.37715115e-03, 1.42980885e-03, 4.33880746e-03,
3.05412428e-03, 1.47275421e-03, 1.64137719e-03, 7.42480172e-04,
7.67868566e-04],
[4.60953083e-04, 7.57756074e-04, 8.82322357e-04, 4.64370677e-05,
2.64032001e-04, 1.36031928e-03, 1.17741674e-04, 8.05659435e-04,
1.12712697e-03, 1.12726433e-03, 6.84354795e-05, 5.84512517e-05,
6.43167162e-04, 2.32909502e-04, 1.89794647e-04, 1.16183962e-03,
7.76742995e-04, 3.42364544e-04, 2.91442379e-04, 1.83797701e-03,
4.33671663e-04],
[4.64462031e-04, 6.01709637e-06, 3.62327007e-04, 2.44055959e-04,
3.80722798e-04, 2.37705067e-04, 4.01768218e-04, 4.17011347e-04,
...
1.11095138e-03, 1.41661755e-04, 1.70812899e-04, 9.95550999e-05,
2.83714845e-04],
[5.16888681e-04, 1.70354216e-04, 2.14118515e-04, 5.10481581e-05,
1.49944180e-04, 1.70695099e-04, 4.94393477e-04, 8.93534280e-05,
5.39842182e-04, 1.38691601e-03, 5.69297256e-05, 8.26071042e-05,
1.11349285e-04, 1.28926148e-04, 5.08707440e-05, 2.73543104e-04,
6.54749470e-04, 1.61615082e-05, 2.30005168e-04, 2.40995402e-04,
1.28346746e-04],
[1.28127636e-04, 1.48374130e-04, 7.36876051e-05, 2.35398700e-04,
7.47705421e-04, 4.37864949e-05, 1.63964594e-04, 1.73373331e-04,
2.07126293e-04, 6.98486954e-05, 4.86252807e-05, 4.32442391e-04,
4.85609546e-04, 3.26246975e-04, 1.98445218e-04, 6.37773215e-05,
3.44230344e-04, 3.07861123e-04, 1.88144883e-05, 5.11511060e-06,
1.00155341e-04],
[9.35751402e-05, 4.52634926e-04, 6.60347511e-05, 1.01850054e-04,
2.69079882e-04, 1.44148578e-04, 3.47006042e-06, 2.43341358e-04,
1.90731161e-04, 1.64827549e-04, 2.29285163e-05, 8.66081727e-05,
5.71547310e-05, 2.38952656e-04, 4.66312730e-05, 6.24048396e-05,
1.33667694e-04, 9.34240916e-05, 7.89594640e-05, 3.09491377e-04,
1.09553161e-04]])
Coordinates:
* frequency (frequency) float64 168B -0.05556 -0.05 ... 0.05 0.05556
* zonalwavenumber (zonalwavenumber) float64 88B 0.0 1.0 2.0 ... 8.0 9.0 10.0
OEE.shape: (11, 21)
ewr: 2.812510787456349
east power: 0.0012679510254192017
west power: 0.0004508253021016655
type(segment_year) <class 'xarray.core.dataarray.DataArray'>
segment_year.shape: (180, 180, 360)
type(daSeaCyc) <class 'xarray.core.dataarray.DataArray'>
daSeaCyc.shape: (180, 180, 360)
type(segment_ano_year) <class 'xarray.core.dataarray.DataArray'>
segment_ano_year.shape: (180, 180, 360)
INFO::2025-07-17 13:28::pcmdi_metrics:: Results saved to a json file: /Users/lee1043/Documents/Research/git/pcmdi_metrics_20230620_pcmdi/pcmdi_metrics/doc/jupyter/Demo/demo_output/mjo/Ex1/mjo_stat_cmip5_historical_da_atm_obs_GPCP-IP_2000-2002.json
2025-07-17 13:28:16,050 [INFO]: base.py(write:429) >> Results saved to a json file: /Users/lee1043/Documents/Research/git/pcmdi_metrics_20230620_pcmdi/pcmdi_metrics/doc/jupyter/Demo/demo_output/mjo/Ex1/mjo_stat_cmip5_historical_da_atm_obs_GPCP-IP_2000-2002.json
2025-07-17 13:28:16,050 [INFO]: base.py(write:429) >> Results saved to a json file: /Users/lee1043/Documents/Research/git/pcmdi_metrics_20230620_pcmdi/pcmdi_metrics/doc/jupyter/Demo/demo_output/mjo/Ex1/mjo_stat_cmip5_historical_da_atm_obs_GPCP-IP_2000-2002.json
INFO::2025-07-17 13:28::pcmdi_metrics:: Results saved to a json file: /Users/lee1043/Documents/Research/git/pcmdi_metrics_20230620_pcmdi/pcmdi_metrics/doc/jupyter/Demo/demo_output/mjo/Ex1/mjo_stat_cmip5_historical_da_atm_2000-2002.json
2025-07-17 13:28:20,186 [INFO]: base.py(write:429) >> Results saved to a json file: /Users/lee1043/Documents/Research/git/pcmdi_metrics_20230620_pcmdi/pcmdi_metrics/doc/jupyter/Demo/demo_output/mjo/Ex1/mjo_stat_cmip5_historical_da_atm_2000-2002.json
2025-07-17 13:28:20,186 [INFO]: base.py(write:429) >> Results saved to a json file: /Users/lee1043/Documents/Research/git/pcmdi_metrics_20230620_pcmdi/pcmdi_metrics/doc/jupyter/Demo/demo_output/mjo/Ex1/mjo_stat_cmip5_historical_da_atm_2000-2002.json
Done
----- GISS-E2-H ---------------------
debug: model_path_list: ['demo_data/CMIP5_demo_timeseries/historical/atmos/day/pr/pr_day_GISS-E2-H_historical_r6i1p1_20000101-20051231.nc']
--- r6i1p1 ---
demo_data/CMIP5_demo_timeseries/historical/atmos/day/pr/pr_day_GISS-E2-H_historical_r6i1p1_20000101-20051231.nc
-- NDJFMA --
debug: open file: demo_data/CMIP5_demo_timeseries/historical/atmos/day/pr/pr_day_GISS-E2-H_historical_r6i1p1_20000101-20051231.nc
debug: check time
debug: startYear, endYear: 2000 2002
debug: NL, NT: 144 180
debug: before year loop: daSeaCyc.shape: (180, 90, 144)
2000
Converting units by multiply 86400.0
debug: year, segment[year][data_var].shape: 2000 (180, 90, 144)
2001
Converting units by multiply 86400.0
debug: year, segment[year][data_var].shape: 2001 (180, 90, 144)
debug: after year loop: daSeaCyc.shape: (180, 90, 144)
debug: year loop start
chk: year: 2000
debug: ds_regrid_subset[data_var] shape: (180, 8, 144)
debug: compute space-time spectrum
chk: year: 2001
debug: ds_regrid_subset[data_var] shape: (180, 8, 144)
debug: compute space-time spectrum
OEE: <xarray.DataArray 'power' (zonalwavenumber: 11, frequency: 21)> Size: 2kB
array([[3.64319816e-05, 3.37420858e-04, 2.95078675e-04, 9.16697570e-04,
1.07091689e-03, 1.23164482e-03, 1.38142241e-03, 5.14193957e-04,
1.72918485e-03, 7.65733145e-03, 1.54544576e-04, 7.65733145e-03,
1.72918485e-03, 5.14193957e-04, 1.38142241e-03, 1.23164482e-03,
1.07091689e-03, 9.16697570e-04, 2.95078675e-04, 3.37420858e-04,
3.64319816e-05],
[1.39043127e-04, 1.85152594e-03, 3.16411192e-03, 1.73217210e-03,
4.21113344e-04, 2.74805560e-04, 1.06323409e-03, 1.22496443e-04,
1.48164150e-03, 1.09625193e-02, 2.97348555e-05, 5.54382260e-03,
2.71550780e-03, 2.83996453e-03, 4.11519437e-03, 2.65601893e-04,
4.38389459e-03, 4.11908474e-04, 2.09992722e-03, 7.16762947e-04,
1.14205061e-03],
[2.20180618e-03, 2.73511219e-03, 8.86673350e-03, 8.37812243e-03,
3.63450908e-04, 6.09409997e-04, 9.64793794e-04, 3.37225106e-04,
1.02106816e-04, 5.87319414e-04, 1.20764384e-04, 3.16798147e-03,
8.04084997e-03, 1.04334949e-03, 7.55838016e-03, 4.34397875e-03,
2.16041922e-03, 6.58014443e-03, 1.29324356e-05, 8.99754470e-05,
5.52778369e-04],
[1.03445823e-03, 1.92229054e-03, 1.40507658e-03, 9.80597348e-04,
1.51172005e-03, 8.07226062e-04, 8.86107293e-04, 1.03368275e-03,
...
5.94729882e-04, 4.24889269e-04, 7.61994589e-05, 7.02075484e-04,
1.72408312e-04],
[3.88845384e-03, 3.17809832e-04, 1.72152570e-04, 5.28006673e-05,
7.05270989e-05, 7.09540836e-04, 1.16077505e-03, 4.45690878e-04,
1.17614581e-05, 3.64360709e-04, 5.52244285e-05, 9.42342347e-05,
3.09261901e-04, 2.64440365e-04, 1.03507038e-03, 1.51162575e-03,
2.07837991e-04, 6.07337165e-04, 6.47632070e-04, 7.06055792e-04,
3.62166302e-04],
[1.54161366e-03, 1.49388989e-04, 6.38178028e-04, 9.16953268e-05,
1.67332478e-03, 2.24154272e-04, 3.78731459e-05, 1.03007787e-03,
7.03852990e-04, 1.39480659e-03, 4.70956771e-05, 1.41611172e-03,
2.32190302e-04, 1.44765976e-04, 2.19475692e-04, 1.30925958e-04,
3.64281568e-04, 3.50338376e-04, 8.27878094e-04, 1.03035586e-04,
3.72160810e-04],
[4.59968743e-04, 5.84846707e-04, 2.13454322e-04, 8.07870559e-04,
7.98661357e-06, 1.04922107e-04, 6.20139107e-04, 2.66727915e-04,
9.63400103e-04, 2.53817857e-04, 1.54311969e-04, 1.43822174e-04,
3.87749099e-04, 3.93962551e-04, 3.58110958e-04, 5.82846355e-04,
4.64778496e-05, 8.70967853e-04, 1.12639831e-03, 6.44800222e-04,
3.66262179e-04]])
Coordinates:
* frequency (frequency) float64 168B -0.05556 -0.05 ... 0.05 0.05556
* zonalwavenumber (zonalwavenumber) float64 88B 0.0 1.0 2.0 ... 8.0 9.0 10.0
OEE.shape: (11, 21)
ewr: 4.2728334890682245
east power: 0.0029892975932121857
west power: 0.000699605449372206
type(segment_year) <class 'xarray.core.dataarray.DataArray'>
segment_year.shape: (180, 90, 144)
type(daSeaCyc) <class 'xarray.core.dataarray.DataArray'>
daSeaCyc.shape: (180, 90, 144)
type(segment_ano_year) <class 'xarray.core.dataarray.DataArray'>
segment_ano_year.shape: (180, 90, 144)
-- MJJASO --
debug: open file: demo_data/CMIP5_demo_timeseries/historical/atmos/day/pr/pr_day_GISS-E2-H_historical_r6i1p1_20000101-20051231.nc
debug: check time
debug: startYear, endYear: 2000 2002
debug: NL, NT: 144 180
debug: before year loop: daSeaCyc.shape: (180, 90, 144)
2000
Converting units by multiply 86400.0
debug: year, segment[year][data_var].shape: 2000 (180, 90, 144)
2001
Converting units by multiply 86400.0
debug: year, segment[year][data_var].shape: 2001 (180, 90, 144)
debug: after year loop: daSeaCyc.shape: (180, 90, 144)
debug: year loop start
chk: year: 2000
debug: ds_regrid_subset[data_var] shape: (180, 8, 144)
debug: compute space-time spectrum
chk: year: 2001
debug: ds_regrid_subset[data_var] shape: (180, 8, 144)
debug: compute space-time spectrum
OEE: <xarray.DataArray 'power' (zonalwavenumber: 11, frequency: 21)> Size: 2kB
array([[1.22640244e-03, 1.36440626e-04, 1.83917376e-03, 1.41693460e-04,
1.21516787e-03, 1.58272356e-04, 3.29276909e-04, 6.77696659e-04,
1.43036505e-03, 9.80366539e-04, 2.97552551e-04, 9.80366539e-04,
1.43036505e-03, 6.77696659e-04, 3.29276909e-04, 1.58272356e-04,
1.21516787e-03, 1.41693460e-04, 1.83917376e-03, 1.36440626e-04,
1.22640244e-03],
[1.08054483e-03, 6.04058970e-04, 9.29363005e-04, 5.38312172e-04,
9.94162673e-04, 2.74341850e-04, 2.76644234e-04, 6.16349299e-04,
3.65336174e-03, 3.00137726e-03, 1.89628283e-04, 3.24095767e-03,
4.15661697e-04, 7.38447679e-04, 2.59395073e-03, 6.67446066e-04,
2.11581802e-03, 1.24140724e-03, 1.69108595e-03, 7.02481255e-04,
8.23921596e-04],
[1.48224635e-03, 1.88550313e-03, 1.88201404e-03, 5.57021969e-04,
1.31236599e-03, 2.26937122e-03, 5.52140729e-04, 1.45341395e-03,
1.94052678e-03, 1.76710888e-03, 4.57354481e-04, 2.96689296e-03,
7.34087523e-04, 2.40529334e-04, 3.27150097e-03, 7.35859194e-04,
3.52796940e-04, 2.39352860e-03, 1.72135137e-03, 5.42214895e-03,
7.09735869e-04],
[1.15653301e-03, 1.59530805e-03, 9.67533819e-05, 3.28244796e-04,
6.37742065e-04, 2.01330001e-03, 3.54949947e-04, 2.89426214e-04,
...
5.54584120e-04, 3.42874172e-05, 2.34191861e-04, 1.81999081e-04,
2.76017993e-04],
[6.00773942e-04, 5.13749212e-04, 1.06707085e-03, 1.66886553e-04,
2.88576238e-04, 1.62376844e-03, 8.81216219e-05, 1.54710887e-04,
1.79179240e-04, 4.35810069e-04, 1.60188307e-04, 6.69949147e-04,
6.35032440e-04, 1.01837757e-04, 5.70299207e-04, 7.61163470e-04,
5.33192525e-04, 3.67408549e-04, 2.61489836e-04, 5.80986160e-05,
1.74714176e-04],
[1.48155112e-03, 4.77845977e-04, 7.06030168e-04, 1.68242782e-03,
9.37803709e-04, 2.42044051e-04, 2.47907249e-03, 9.11978902e-04,
8.79143373e-04, 1.33717449e-03, 1.01696568e-04, 1.82385011e-04,
7.73383761e-04, 7.15724453e-04, 8.00130815e-04, 2.08954754e-03,
9.03664676e-04, 3.88019755e-04, 2.15494074e-04, 7.04628316e-05,
3.69811070e-04],
[7.00625886e-05, 1.44776886e-03, 4.77283929e-04, 5.26383910e-04,
6.48947054e-04, 1.61412028e-04, 7.98014063e-04, 3.05455169e-04,
1.59852861e-04, 5.66278867e-04, 8.40366355e-05, 1.15848255e-03,
2.44524965e-03, 3.45062443e-04, 1.75681895e-04, 6.81208605e-05,
1.96062830e-04, 3.74304730e-04, 8.27316567e-04, 9.76322102e-05,
6.81309693e-04]])
Coordinates:
* frequency (frequency) float64 168B -0.05556 -0.05 ... 0.05 0.05556
* zonalwavenumber (zonalwavenumber) float64 88B 0.0 1.0 2.0 ... 8.0 9.0 10.0
OEE.shape: (11, 21)
ewr: 1.1738950081572066
east power: 0.0010803950724325414
west power: 0.0009203506829188733
type(segment_year) <class 'xarray.core.dataarray.DataArray'>
segment_year.shape: (180, 90, 144)
type(daSeaCyc) <class 'xarray.core.dataarray.DataArray'>
daSeaCyc.shape: (180, 90, 144)
type(segment_ano_year) <class 'xarray.core.dataarray.DataArray'>
segment_ano_year.shape: (180, 90, 144)
INFO::2025-07-17 13:28::pcmdi_metrics:: Results saved to a json file: /Users/lee1043/Documents/Research/git/pcmdi_metrics_20230620_pcmdi/pcmdi_metrics/doc/jupyter/Demo/demo_output/mjo/Ex1/mjo_stat_cmip5_historical_da_atm_GISS-E2-H_r6i1p1_2000-2002.json
2025-07-17 13:28:28,763 [INFO]: base.py(write:429) >> Results saved to a json file: /Users/lee1043/Documents/Research/git/pcmdi_metrics_20230620_pcmdi/pcmdi_metrics/doc/jupyter/Demo/demo_output/mjo/Ex1/mjo_stat_cmip5_historical_da_atm_GISS-E2-H_r6i1p1_2000-2002.json
2025-07-17 13:28:28,763 [INFO]: base.py(write:429) >> Results saved to a json file: /Users/lee1043/Documents/Research/git/pcmdi_metrics_20230620_pcmdi/pcmdi_metrics/doc/jupyter/Demo/demo_output/mjo/Ex1/mjo_stat_cmip5_historical_da_atm_GISS-E2-H_r6i1p1_2000-2002.json
INFO::2025-07-17 13:28::pcmdi_metrics:: Results saved to a json file: /Users/lee1043/Documents/Research/git/pcmdi_metrics_20230620_pcmdi/pcmdi_metrics/doc/jupyter/Demo/demo_output/mjo/Ex1/mjo_stat_cmip5_historical_da_atm_2000-2002.json
2025-07-17 13:28:32,844 [INFO]: base.py(write:429) >> Results saved to a json file: /Users/lee1043/Documents/Research/git/pcmdi_metrics_20230620_pcmdi/pcmdi_metrics/doc/jupyter/Demo/demo_output/mjo/Ex1/mjo_stat_cmip5_historical_da_atm_2000-2002.json
2025-07-17 13:28:32,844 [INFO]: base.py(write:429) >> Results saved to a json file: /Users/lee1043/Documents/Research/git/pcmdi_metrics_20230620_pcmdi/pcmdi_metrics/doc/jupyter/Demo/demo_output/mjo/Ex1/mjo_stat_cmip5_historical_da_atm_2000-2002.json
Done
Command line arguments
It is also possible to use the command line to pass other arguments to the MJO driver. The command line arguments will override the settings in the parameter file.
In these example, we change a few different things. The new case_id
will show up as a new folder in the result directory because of how the result_dir
variable is set in the parameter file. NetCDF output and plots will be saved. The analysis start and end years for the models are changed to match the observations.
The includeOBS
flag means that results will also be generated for observations, while the no_OBS
flag excludes observations.
[5]:
%%bash
mjo_metrics_driver.py -p basic_mjo_param.py \
--case_id 'Ex2' \
--no_OBS \
--nc_out True \
--plot True \
--msyear 2000 \
--meyear 2005
includeOBS: False
models: ['GISS-E2-H']
realization: r6i1p1
output directories:
graphics : demo_output/mjo/Ex2
diagnostic_results : demo_output/mjo/Ex2
metrics_results : demo_output/mjo/Ex2
CMEC: False
debug: False
parallel: False
----- GISS-E2-H ---------------------
--- r6i1p1 ---
demo_data/CMIP5_demo_timeseries/historical/atmos/day/pr/pr_day_GISS-E2-H_historical_r6i1p1_20000101-20051231.nc
-- NDJFMA --
2000
Converting units by multiply 86400.0
2001
Converting units by multiply 86400.0
2002
Converting units by multiply 86400.0
2003
Converting units by multiply 86400.0
2004
Converting units by multiply 86400.0
chk: year: 2000
chk: year: 2001
chk: year: 2002
chk: year: 2003
chk: year: 2004
ewr: 1.549148912834605
east power: 0.005931412302887387
west power: 0.0038288199757595897
-- MJJASO --
2000
Converting units by multiply 86400.0
2001
Converting units by multiply 86400.0
2002
Converting units by multiply 86400.0
2003
Converting units by multiply 86400.0
2004
Converting units by multiply 86400.0
chk: year: 2000
chk: year: 2001
chk: year: 2002
chk: year: 2003
chk: year: 2004
ewr: 0.9988487228662595
east power: 0.0024578884945468
west power: 0.002460721466904151
INFO::2025-07-17 13:28::pcmdi_metrics:: Results saved to a json file: /Users/lee1043/Documents/Research/git/pcmdi_metrics_20230620_pcmdi/pcmdi_metrics/doc/jupyter/Demo/demo_output/mjo/Ex2/mjo_stat_cmip5_historical_da_atm_GISS-E2-H_r6i1p1_2000-2005.json
2025-07-17 13:28:48,964 [INFO]: base.py(write:429) >> Results saved to a json file: /Users/lee1043/Documents/Research/git/pcmdi_metrics_20230620_pcmdi/pcmdi_metrics/doc/jupyter/Demo/demo_output/mjo/Ex2/mjo_stat_cmip5_historical_da_atm_GISS-E2-H_r6i1p1_2000-2005.json
2025-07-17 13:28:48,964 [INFO]: base.py(write:429) >> Results saved to a json file: /Users/lee1043/Documents/Research/git/pcmdi_metrics_20230620_pcmdi/pcmdi_metrics/doc/jupyter/Demo/demo_output/mjo/Ex2/mjo_stat_cmip5_historical_da_atm_GISS-E2-H_r6i1p1_2000-2005.json
INFO::2025-07-17 13:28::pcmdi_metrics:: Results saved to a json file: /Users/lee1043/Documents/Research/git/pcmdi_metrics_20230620_pcmdi/pcmdi_metrics/doc/jupyter/Demo/demo_output/mjo/Ex2/mjo_stat_cmip5_historical_da_atm_2000-2005.json
2025-07-17 13:28:53,132 [INFO]: base.py(write:429) >> Results saved to a json file: /Users/lee1043/Documents/Research/git/pcmdi_metrics_20230620_pcmdi/pcmdi_metrics/doc/jupyter/Demo/demo_output/mjo/Ex2/mjo_stat_cmip5_historical_da_atm_2000-2005.json
2025-07-17 13:28:53,132 [INFO]: base.py(write:429) >> Results saved to a json file: /Users/lee1043/Documents/Research/git/pcmdi_metrics_20230620_pcmdi/pcmdi_metrics/doc/jupyter/Demo/demo_output/mjo/Ex2/mjo_stat_cmip5_historical_da_atm_2000-2005.json
Done
A png image is created showing the wavenumber-frequency power spectra.
[6]:
from IPython.display import Image
Image(filename=demo_output_directory+"/mjo/Ex2/cmip5_GISS-E2-H_historical_r6i1p1_mjo_2000-2005_NDJFMA_cmmGrid.png")
[6]:
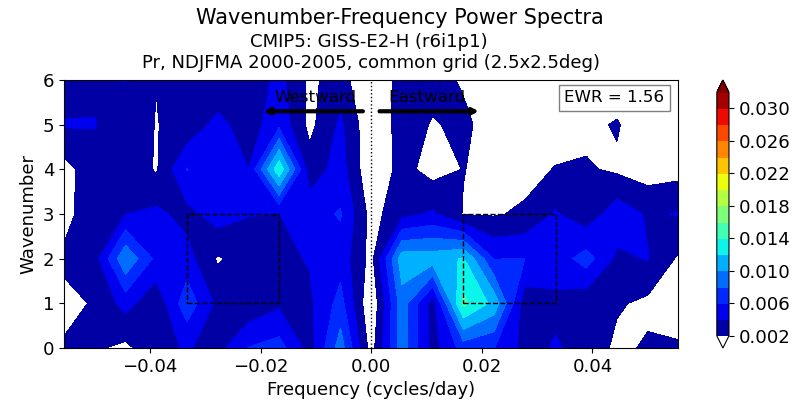